C# Data Types
There are two categories in which C# data types are classified into - 1. Value types and 2. Reference types. The difference is based on the way by which data is stored into the variables. In value types, the variable holds the actual data while in the reference types the variable holds the reference to the data.
Each variable of value type carries its own copy of data while it may be possible that two variables of reference types can refer to the same data and in this case changes made in one variable will reflect the changes on another variable. We will see the value types now.
Value Types
Value types are further categorized into:
- Simple Types (Integer, Floating, Decimal, Character, Bool)
- Enum Types
- Struct Types
- Nullable value types
The Enum, Struct and Nullable value types will be covered later in this course. Let's explore Simple Types for now.
Integer Types : Integers can be signed or unsigned. The difference is signed types carry one more space of storing the negative (-) sign while unsigned does not. Hence unsigned integers are always positive integers. There are 4 types of signed integer types - sbyte (8 bits), short (16 bits), int (32 bits), long (64 bits). Similarly, there are 4 types of unsigned integer types - byte (8 bits), ushort (16 bits), uint (32 bits), ulong (64 bits).
Character Types : C# uses Unicode to define and store a character. To declare a character we use the "char" keyword.
Floating Point Types : These are the numbers that are fractional. C# supports two types of floating point types: float and double. The only difference is that the float is 32 bits precision range while double has a 64 bits precision range.
Decimal Type : There is one type in C# which is defined by the keyword "decimal" and it represents 128 bits wide precision. It is also different from the floating point by the fact that it can precisely handle the arithmetic operations without losing precision up to 28 decimal points. That's why it is commonly used in financial calculations.
Bool Type : This is defined by the keyword "bool" and it represents only two values "true" and "false" which is equivalent to integer type values 1 and 0.
The reference types includes Classes, Arrays, Delegates and Interfaces. Each of these will be covered later in this course.
C# Variables
A variable is C# holds the value of one of the data types in C#. A variable in C# is declared with the syntax -
<type> <var-name>;
e.g. :
int age;
To initialize a declared variable we assign a value to it
age = 10;
A variable can be declared and initialized at the same time with this syntax -
type var-name = value;
e.g. :
int age = 10;
Implicitly typed variables
We can declare a variable without mentioning its data type and in this case we need to initialize the variable at the time of declaration. We do this by using "var" keyword. e.g. -
var age = 10;
The compiler infers the data type of the variable by the value that is being assigned to the variable. In the above example, the compiler check the value "10" and it infers that the data type of the value 10 must be integer. When we compile the program, the compiler replaces the keyword "var" with "System.Int32" in MSIL (Remember Common Type System from previous chapter, System.Int32 is the equivalent of int in C#). Once the value is assigned first time, we can not assign any other value of different data type. This compiler will show error in this case.
Consider the below example, the compiler will show an error because we are trying to re-assign a different data type value which is string in this case.
var age = 10;
age = "ten";
Dynamic variables
With "dynamic" keyword, we can make any variable dynamic. By dynamic variable we mean that unlike "var", any value of any data type can be re-assigned to the variable.
Consider the below example, the compiler will not show any error.
dynamic age = 10;
age = "ten";
The problem with the dynamic variable is it becomes the responsibility of the developer to take care of type compatibilities because incompatible operations on the dynamic variable will throw an exception on runtime. For e.g. the following lines of code will now give error at compile time but will throw error at runtime.
using System;
class Program
{
static void Main(string[] args)
{
dynamic age = 10;
Console.WriteLine(age / "20");
Console.Write("Press any key to continue...");
Console.ReadKey(true);
}
}
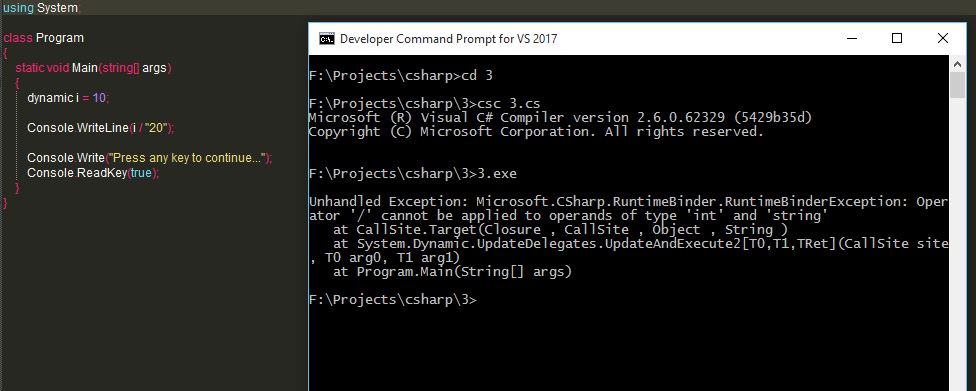
Automatic data type conversion
Consider the following lines of code
using System;
class Program
{
static void Main(string[] args)
{
long width = 100;
double length = width * 2;
Console.Write("Press any key to continue...");
Console.ReadKey(true);
}
}
Even though, the variable "width" and "length" have different data types, the compiler gives you no error. The reason is there is automatic type conversion taking place in the expression "double length = width * 2". However, this is not always be the case. It is possible only in case of the below two conditions.
- Both the types are compatible.
- The range of data type of the destination variable must be greater than the range supported by the source variable's data type. In the above example, since "double" has a much wider range than "long", an automatic conversion takes place at compile time.
Data type casting
Now, what if we want to use a variable with a larger range than the destination variable's data type. Well, C# provides us with a way to do that by means of Casting. Let's consider the following example.
using System;
class Program
{
static void Main(string[] args)
{
double width = 100;
long length = width * 2;
Console.Write("Press any key to continue...");
Console.ReadKey(true);
}
}
It will show the following compile time error.
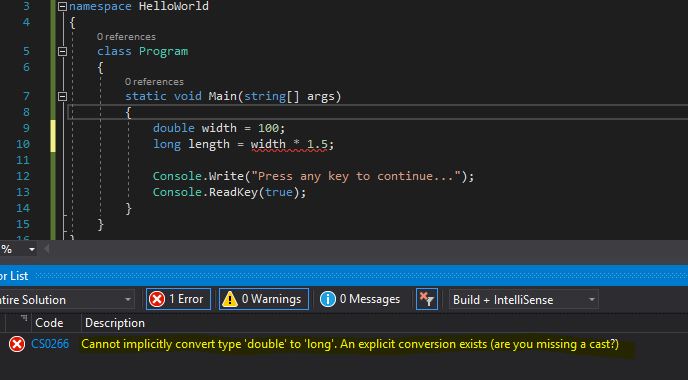
What we need now is Casting and following is the code which is showing how to do that.
using System;
class Program
{
static void Main(string[] args)
{
double width = 100;
long length = (long)(width) * 2;
Console.Write("Press any key to continue...");
Console.ReadKey(true);
}
}
In the above example, we have casted the the variable "width" to convert it into "long" data type. You can copy paste the above code in Visual Studio and check yourself. Following is the general syntax for casting a variable -
(<data-type>)<variable-name>