What is Angular?
Angular is a complete framework for building client application or primarily browser based applications. Angular allows us to write client application in Component Based Design Pattern. It offers us to write both in Javascript and Typescript, however it majorly prefers TypeScript.
So, now you know with Angular we build browser based applications. To explain this further let's first understand the thought process around Angular.
Component Design - The Angular's way of developing an application
A client/browser application can be simply build using HTML, CSS and Javascript. Problem occurs when the application starts growing it becomes hard and hard to maintain and incorporating/integrating changes.
Angular solves this problem by providing a Component Based Design Pattern . This is a pattern where we first figure out various components of the application, take each component in centre and build application around it.
The benefit of using Component Based Design is the we can reuse different components at different places in our application. A Component can be a small or big part of the application. A Component can contain other components.
A Component design approach is very useful from UI/UX perspective because if we see things in real world we realize that those are individual entities or are made up of some other entities. For e.g. an air conditioner consists of a fan, a condenser, electric assemblies etc. So, an AC is also a Component which we fit in our home (app) and it is made up of several other components.
Now since I took a real world example, the question arises that what is the difference between Component Based Design and Object Oriented Design. This is very important to understand.
Object Oriented Design v/s Component Based Design
In context of building client/browser based application, the Object Oriented Design is all about writing model classes and providing functionality to them. This we usually achieve be making classes and objects javascript only and use this over html and css.
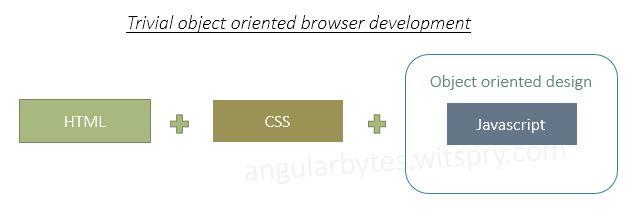
In a Component Based Design we bother about creating a complete unit or piece which wraps HTML, CSS and Javascript together which will be enough to execute or display that unit. An application is a collection of components and a component can contain several other components.
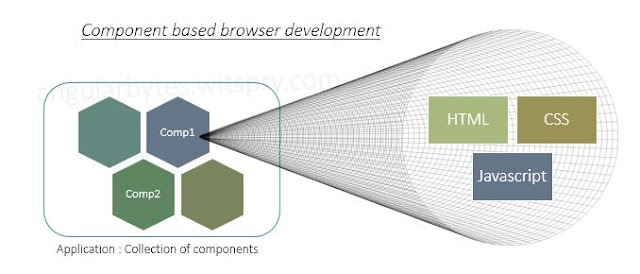
How a Component in Angular looks like?
In Angular, we use to write component classes. Each Component class contains an HTML templates and logics to operate on that template.
import { Component } from '@angular/core';
export class Player {
id: number;
name: string;
}
@Component({
selector: 'my-app',
template: `
<h1></h1>
<h2> details!</h2>
<div><label>id: </label></div>
<div>
<label>name: </label>
<input [(ngModel)]="player.name" placeholder="name">
</div>
`
})
export class AppComponent {
title = 'Chess Tournament';
player: Player = {
id: 1,
name: 'Jeff'
};
}
As you can see there is a model class Player having two properties id and name. Also, there is one html template where some properties have been mentioned under different brackets. There is one AppComponent class where properties data has been assigned to them.
Don't worry about the code at this point of time, you will learn gradually as you will progress through this course.
Angular Architecture
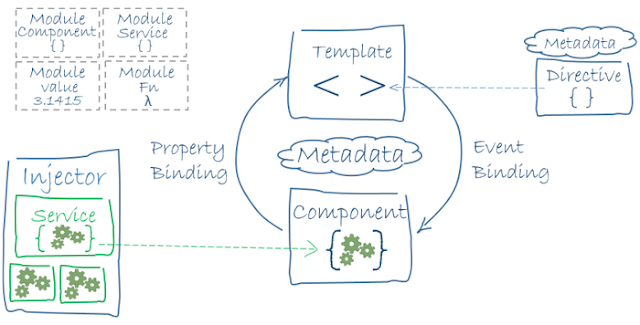
A single unit of application in Angular is called Module . A module consists of different components, services and functions. Angular's own module is called ngModule which should be imported in the application before using Angular and its features.
Each Component class is associated with metadata. In the above example we have selector and template as a part of Angular metadata.
Angular also provides bindings to bind component class property data to the template.
Angular's provides inbuilt and custom services that can be injected in any component to make use of service functionalities.
So let's begin developing with Angular.